saysynth.core.lyrics
The Lyric class enables "singing"-like speech synthesis
by translating input text into a list of phonemes which can
mapped onto musical passages through other saysynth
functions.
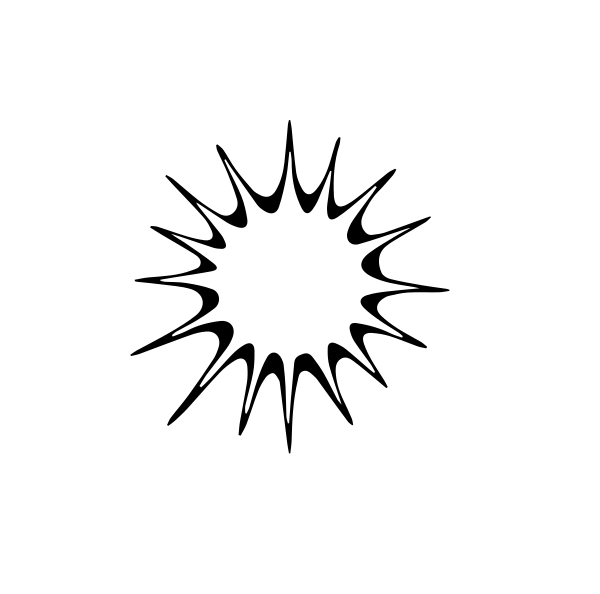
1""" 2The Lyric class enables "singing"-like speech synthesis 3by translating input text into a list of phonemes which can 4mapped onto musical passages through other `saysynth` functions. 5<center><img src="/assets/img/cell.png"></img></center> 6""" 7from functools import cached_property 8from typing import List 9 10from ..lib import nlp 11from .word import Word 12 13 14class Lyrics(object): 15 def __init__(self, text: str): 16 """ 17 The Lyric class enables "singing"-like speech synthesis 18 by translating input text into a list of phonemes which can 19 mapped onto musical passages through other `saysynth` functions. 20 Args: 21 text: The text of the lyrics. Use punctuation for pauses. 22 """ 23 self.text = text 24 25 def get_phoneme_for_index(self, index: int) -> str: 26 """ 27 Given an index (for instance in an iteration) return 28 a phoneme at the respective position. This operation 29 uses a modulo such that a text is repeated over and 30 over until the full musical passage has been generated. 31 """ 32 seg_index = index % self.n_phonemes 33 return self.phonemes[seg_index] 34 35 def get_phonemes(self, start_at: int = 0, end_at: int = -1) -> List[str]: 36 """ 37 Get phonemes of these lyrics between a specific index range. 38 """ 39 return self.phonemes[start_at:end_at] 40 41 @cached_property 42 def words(self) -> List[Word]: 43 """ 44 A list of `Word` objects in the text. 45 """ 46 return [Word(*word) for word in nlp.process_text_for_say(self.text)] 47 48 @cached_property 49 def n_words(self) -> int: 50 """ 51 The number of syllables in the text. 52 """ 53 return len(self.words) 54 55 @cached_property 56 def n_syllables(self) -> int: 57 """ 58 The number of syllables in the text. 59 """ 60 return sum([word.syllables for word in self.words]) 61 62 @cached_property 63 def phonemes(self) -> List[str]: 64 """ 65 The list of phonemes in the text. 66 """ 67 return [p for word in self.words for p in word.phonemes] 68 69 @cached_property 70 def n_phonemes(self) -> int: 71 """ 72 The number of phonemes in the text. 73 """ 74 return len(self.phonemes)
class
Lyrics:
15class Lyrics(object): 16 def __init__(self, text: str): 17 """ 18 The Lyric class enables "singing"-like speech synthesis 19 by translating input text into a list of phonemes which can 20 mapped onto musical passages through other `saysynth` functions. 21 Args: 22 text: The text of the lyrics. Use punctuation for pauses. 23 """ 24 self.text = text 25 26 def get_phoneme_for_index(self, index: int) -> str: 27 """ 28 Given an index (for instance in an iteration) return 29 a phoneme at the respective position. This operation 30 uses a modulo such that a text is repeated over and 31 over until the full musical passage has been generated. 32 """ 33 seg_index = index % self.n_phonemes 34 return self.phonemes[seg_index] 35 36 def get_phonemes(self, start_at: int = 0, end_at: int = -1) -> List[str]: 37 """ 38 Get phonemes of these lyrics between a specific index range. 39 """ 40 return self.phonemes[start_at:end_at] 41 42 @cached_property 43 def words(self) -> List[Word]: 44 """ 45 A list of `Word` objects in the text. 46 """ 47 return [Word(*word) for word in nlp.process_text_for_say(self.text)] 48 49 @cached_property 50 def n_words(self) -> int: 51 """ 52 The number of syllables in the text. 53 """ 54 return len(self.words) 55 56 @cached_property 57 def n_syllables(self) -> int: 58 """ 59 The number of syllables in the text. 60 """ 61 return sum([word.syllables for word in self.words]) 62 63 @cached_property 64 def phonemes(self) -> List[str]: 65 """ 66 The list of phonemes in the text. 67 """ 68 return [p for word in self.words for p in word.phonemes] 69 70 @cached_property 71 def n_phonemes(self) -> int: 72 """ 73 The number of phonemes in the text. 74 """ 75 return len(self.phonemes)
Lyrics(text: str)
16 def __init__(self, text: str): 17 """ 18 The Lyric class enables "singing"-like speech synthesis 19 by translating input text into a list of phonemes which can 20 mapped onto musical passages through other `saysynth` functions. 21 Args: 22 text: The text of the lyrics. Use punctuation for pauses. 23 """ 24 self.text = text
The Lyric class enables "singing"-like speech synthesis
by translating input text into a list of phonemes which can
mapped onto musical passages through other saysynth
functions.
Arguments:
- text: The text of the lyrics. Use punctuation for pauses.
def
get_phoneme_for_index(self, index: int) -> str:
26 def get_phoneme_for_index(self, index: int) -> str: 27 """ 28 Given an index (for instance in an iteration) return 29 a phoneme at the respective position. This operation 30 uses a modulo such that a text is repeated over and 31 over until the full musical passage has been generated. 32 """ 33 seg_index = index % self.n_phonemes 34 return self.phonemes[seg_index]
Given an index (for instance in an iteration) return a phoneme at the respective position. This operation uses a modulo such that a text is repeated over and over until the full musical passage has been generated.
def
get_phonemes(self, start_at: int = 0, end_at: int = -1) -> List[str]:
36 def get_phonemes(self, start_at: int = 0, end_at: int = -1) -> List[str]: 37 """ 38 Get phonemes of these lyrics between a specific index range. 39 """ 40 return self.phonemes[start_at:end_at]
Get phonemes of these lyrics between a specific index range.