saysynth.core.word
The Word class contains info on the text, number of syllables,
and list of phonemes in a word. It's used in the Lyric
class.
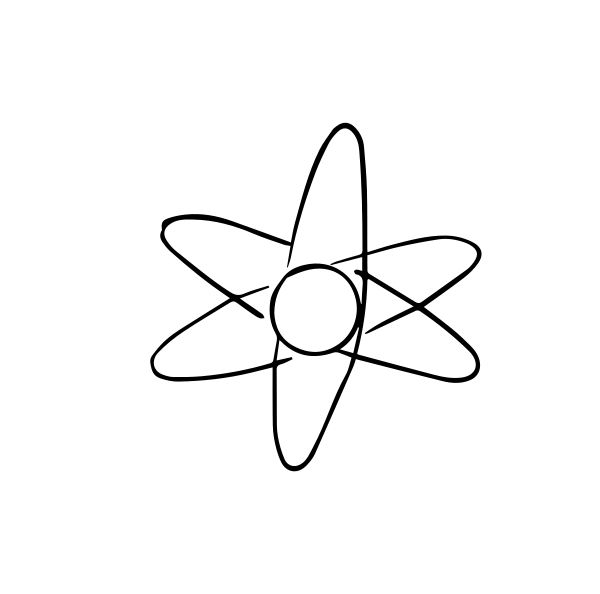
1""" 2The Word class contains info on the text, number of syllables, 3and list of phonemes in a word. It's used in the `Lyric` class. 4 5<center><img src="/assets/img/nuclear.png"></img></center> 6""" 7import math 8from typing import List 9 10 11class Word(object): 12 def __init__(self, text: str, syllables: int, phonemes: List[str]): 13 """ 14 The Word class contains info on the text, number of syllables, 15 and list of phonemes in a word. It's used in the `Lyric` class. 16 Args: 17 text: The raw text of the word 18 syllables: The number of syllables in the word 19 phonemes: The list of phonemes in the word 20 """ 21 self.text = text 22 self.syllables = syllables 23 self.phonemes = phonemes 24 self.n_phonemes = len(self.phonemes) 25 self.max_phonemes_per_syllable = math.floor( 26 self.n_phonemes / self.syllables 27 )
class
Word:
12class Word(object): 13 def __init__(self, text: str, syllables: int, phonemes: List[str]): 14 """ 15 The Word class contains info on the text, number of syllables, 16 and list of phonemes in a word. It's used in the `Lyric` class. 17 Args: 18 text: The raw text of the word 19 syllables: The number of syllables in the word 20 phonemes: The list of phonemes in the word 21 """ 22 self.text = text 23 self.syllables = syllables 24 self.phonemes = phonemes 25 self.n_phonemes = len(self.phonemes) 26 self.max_phonemes_per_syllable = math.floor( 27 self.n_phonemes / self.syllables 28 )
Word(text: str, syllables: int, phonemes: List[str])
13 def __init__(self, text: str, syllables: int, phonemes: List[str]): 14 """ 15 The Word class contains info on the text, number of syllables, 16 and list of phonemes in a word. It's used in the `Lyric` class. 17 Args: 18 text: The raw text of the word 19 syllables: The number of syllables in the word 20 phonemes: The list of phonemes in the word 21 """ 22 self.text = text 23 self.syllables = syllables 24 self.phonemes = phonemes 25 self.n_phonemes = len(self.phonemes) 26 self.max_phonemes_per_syllable = math.floor( 27 self.n_phonemes / self.syllables 28 )
The Word class contains info on the text, number of syllables,
and list of phonemes in a word. It's used in the Lyric
class.
Arguments:
- text: The raw text of the word
- syllables: The number of syllables in the word
- phonemes: The list of phonemes in the word