saysynth.cli
The saysynth.cli
module contains submodules for all saysynth
subcommands, including any shared options
.
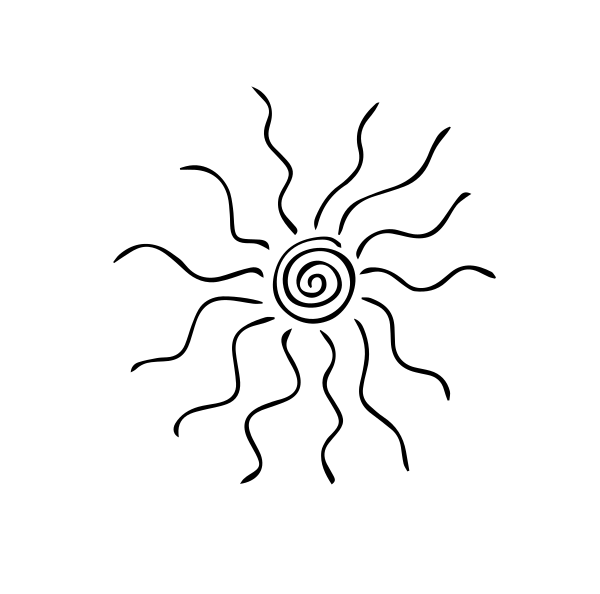
1""" 2The `saysynth.cli` module contains submodules for all `saysynth` subcommands, including any shared `options`. 3 4<center><img src="/assets/img/sun-wavy.png"></img></center> 5""" 6 7import os 8 9import click 10 11COMMAND_METHOD = "cli" 12COMMANDS_FOLDER = os.path.join(os.path.dirname(__file__), "commands") 13COMMAND_NAMES = [ 14 filename[:-3] 15 for filename in os.listdir(COMMANDS_FOLDER) 16 if filename.endswith(".py") and not filename.startswith("__") 17] 18 19 20class SaySynthCLI(click.MultiCommand): 21 """ 22 The SaySynthCLI dynamically loads functions from the 23 `saysynth.cli.commands` module. 24 """ 25 26 commands = COMMAND_NAMES 27 28 def list_commands(self, ctx): 29 return self.commands 30 31 def get_command(self, ctx, name): 32 if name not in self.commands: 33 raise ValueError(f"Invalid command name: {name}") 34 ns = {} 35 fn = os.path.join(COMMANDS_FOLDER, name + ".py") 36 with open(fn) as f: 37 code = compile(f.read(), fn, "exec") 38 eval(code, ns, ns) 39 return ns[COMMAND_METHOD] 40 41 42main = SaySynthCLI(help="Make music with Mac's say command.") 43 44if __name__ == "__main__": 45 main()
class
SaySynthCLI(click.core.MultiCommand):
21class SaySynthCLI(click.MultiCommand): 22 """ 23 The SaySynthCLI dynamically loads functions from the 24 `saysynth.cli.commands` module. 25 """ 26 27 commands = COMMAND_NAMES 28 29 def list_commands(self, ctx): 30 return self.commands 31 32 def get_command(self, ctx, name): 33 if name not in self.commands: 34 raise ValueError(f"Invalid command name: {name}") 35 ns = {} 36 fn = os.path.join(COMMANDS_FOLDER, name + ".py") 37 with open(fn) as f: 38 code = compile(f.read(), fn, "exec") 39 eval(code, ns, ns) 40 return ns[COMMAND_METHOD]
The SaySynthCLI dynamically loads functions from the
saysynth.cli.commands
module.
def
get_command(self, ctx, name):
32 def get_command(self, ctx, name): 33 if name not in self.commands: 34 raise ValueError(f"Invalid command name: {name}") 35 ns = {} 36 fn = os.path.join(COMMANDS_FOLDER, name + ".py") 37 with open(fn) as f: 38 code = compile(f.read(), fn, "exec") 39 eval(code, ns, ns) 40 return ns[COMMAND_METHOD]
Given a context and a command name, this returns a
Command
object if it exists or returns None
.
Inherited Members
- click.core.MultiCommand
- MultiCommand
- to_info_dict
- collect_usage_pieces
- format_options
- result_callback
- format_commands
- parse_args
- invoke
- resolve_command
- shell_complete
- click.core.Command
- get_usage
- get_params
- format_usage
- get_help_option_names
- get_help_option
- make_parser
- get_help
- get_short_help_str
- format_help
- format_help_text
- format_epilog
- click.core.BaseCommand
- make_context
- main