saysynth.core.base
The SayObject
class provides standard runtime functionality to monotonic features like arp
, note
, and midi_track
.
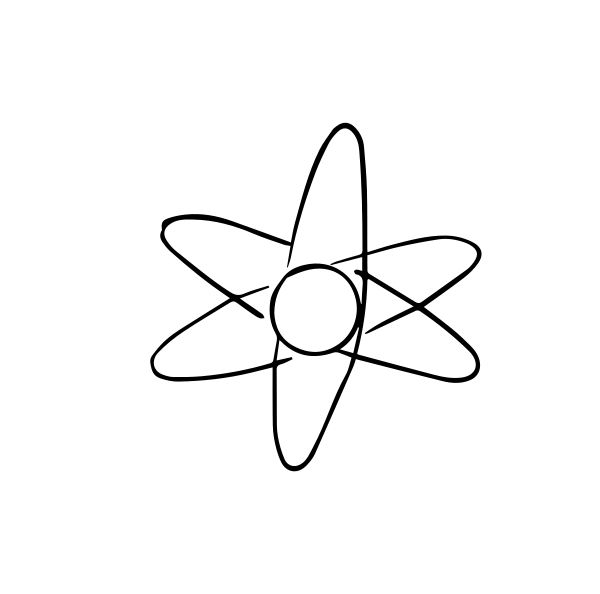
1""" 2The `SayObject` class provides standard runtime functionality to monotonic features like `arp`, `note`, and `midi_track`. 3<center><img src="/assets/img/nuclear.png"></img></center> 4""" 5import sys 6 7from ..cli.options import prepare_options_for_say 8from ..constants import SAY_TUNE_TAG 9from ..lib import say 10 11 12class SayObject(object): 13 def to_text(self): 14 """Render this object as Apple SpeechSynthesis DSL text""" 15 raise NotImplementedError 16 17 def to_say_text(self): 18 """ 19 Render this object as Apple SpeechSynthesis DSL text, 20 including the initial [[TUNE]] tag. 21 """ 22 return SAY_TUNE_TAG + "\n" + self.to_text() 23 24 def write(self, output_file) -> None: 25 """ 26 Render this Arp as Apple SpeechSynthesis DSL text, 27 and write it to an output file 28 """ 29 with open(output_file, "w") as f: 30 f.write(self.to_say_text()) 31 32 def play(self, **say_options) -> None: 33 """ 34 Play this object and pass in additional options to `say.run` 35 """ 36 text = self.to_say_text() 37 options = prepare_options_for_say(text, **say_options) 38 say.run(**options) 39 40 @classmethod 41 def new(cls, **kwargs): 42 """ 43 Instantiate this object. 44 """ 45 return cls(**kwargs) 46 47 def cli(self, **kwargs) -> None: 48 """ 49 Handle the execution of this object 50 within the context of the CLI. 51 """ 52 klass = self.new(**kwargs) 53 output_file = kwargs.get("output_file") 54 if output_file: 55 klass.write(output_file) 56 elif kwargs.get("no_exec", False): 57 sys.stdout.write(klass.to_say_text()) 58 else: 59 klass.play(**kwargs)
class
SayObject:
13class SayObject(object): 14 def to_text(self): 15 """Render this object as Apple SpeechSynthesis DSL text""" 16 raise NotImplementedError 17 18 def to_say_text(self): 19 """ 20 Render this object as Apple SpeechSynthesis DSL text, 21 including the initial [[TUNE]] tag. 22 """ 23 return SAY_TUNE_TAG + "\n" + self.to_text() 24 25 def write(self, output_file) -> None: 26 """ 27 Render this Arp as Apple SpeechSynthesis DSL text, 28 and write it to an output file 29 """ 30 with open(output_file, "w") as f: 31 f.write(self.to_say_text()) 32 33 def play(self, **say_options) -> None: 34 """ 35 Play this object and pass in additional options to `say.run` 36 """ 37 text = self.to_say_text() 38 options = prepare_options_for_say(text, **say_options) 39 say.run(**options) 40 41 @classmethod 42 def new(cls, **kwargs): 43 """ 44 Instantiate this object. 45 """ 46 return cls(**kwargs) 47 48 def cli(self, **kwargs) -> None: 49 """ 50 Handle the execution of this object 51 within the context of the CLI. 52 """ 53 klass = self.new(**kwargs) 54 output_file = kwargs.get("output_file") 55 if output_file: 56 klass.write(output_file) 57 elif kwargs.get("no_exec", False): 58 sys.stdout.write(klass.to_say_text()) 59 else: 60 klass.play(**kwargs)
def
to_text(self):
14 def to_text(self): 15 """Render this object as Apple SpeechSynthesis DSL text""" 16 raise NotImplementedError
Render this object as Apple SpeechSynthesis DSL text
def
to_say_text(self):
18 def to_say_text(self): 19 """ 20 Render this object as Apple SpeechSynthesis DSL text, 21 including the initial [[TUNE]] tag. 22 """ 23 return SAY_TUNE_TAG + "\n" + self.to_text()
Render this object as Apple SpeechSynthesis DSL text, including the initial [[TUNE]] tag.
def
write(self, output_file) -> None:
25 def write(self, output_file) -> None: 26 """ 27 Render this Arp as Apple SpeechSynthesis DSL text, 28 and write it to an output file 29 """ 30 with open(output_file, "w") as f: 31 f.write(self.to_say_text())
Render this Arp as Apple SpeechSynthesis DSL text, and write it to an output file
def
play(self, **say_options) -> None:
33 def play(self, **say_options) -> None: 34 """ 35 Play this object and pass in additional options to `say.run` 36 """ 37 text = self.to_say_text() 38 options = prepare_options_for_say(text, **say_options) 39 say.run(**options)
Play this object and pass in additional options to say.run
@classmethod
def
new(cls, **kwargs):
41 @classmethod 42 def new(cls, **kwargs): 43 """ 44 Instantiate this object. 45 """ 46 return cls(**kwargs)
Instantiate this object.
def
cli(self, **kwargs) -> None:
48 def cli(self, **kwargs) -> None: 49 """ 50 Handle the execution of this object 51 within the context of the CLI. 52 """ 53 klass = self.new(**kwargs) 54 output_file = kwargs.get("output_file") 55 if output_file: 56 klass.write(output_file) 57 elif kwargs.get("no_exec", False): 58 sys.stdout.write(klass.to_say_text()) 59 else: 60 klass.play(**kwargs)
Handle the execution of this object within the context of the CLI.